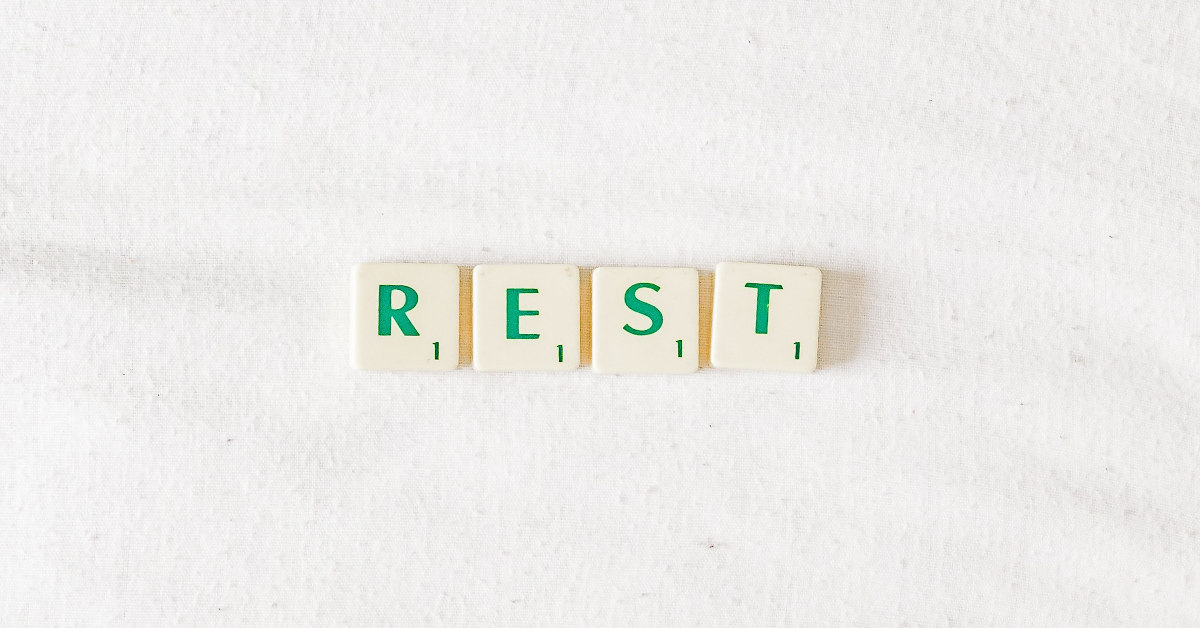
REST APIs for Product Managers
Early on as a product manager in tech, it was clear to me that a functional understanding of APIs is vital for anyone working as an intermediary between developers and stakeholders. In seeking to learn more, I found that many resources were either too technical or didn’t dive deep enough. In this article, I hope to find the Goldilocks zone; I don’t want to talk about the technical implementation of an API integration, but I will provide enough foundational knowledge for the layman to speak with confidence.
What is an API?
It’s a quick three-letter acronym that gets thrown around so much, you may feel afraid to ask. Fear no more! API stands for Application Programming Interface. At it’s most basic level, an API is a piece of software that allows two applications to communicate. They do so through a basic model that emulates a conversation – one party initiates a request, and the other issues a response. Through this article, I’ll specifically talk about a variety of API called a REST API.
Imagine walking into a new pizzeria and inquiring about the cost of a slice of (clearly superior New York style) pizza. You issue a request like “Hello, how much is a slice of cheese pizza?” The worker behind the counter issues a response such as “One slice of cheese pizza costs $2.50”. In this example, you’ve provided enough information for the worker to process your request, and in return you get a response containing the state of the resources you requested, or an error, if something goes wrong. That’s exactly how an API works!
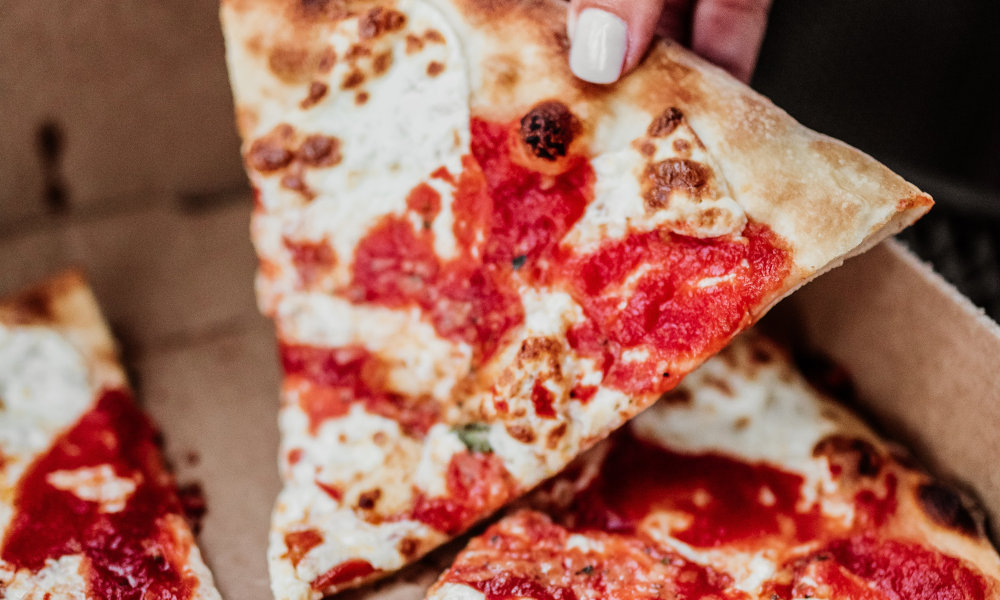
Let’s apply that idea to an API now. Say I’m developing an app for my computer desktop that displays the weather at my location. I don’t have the means or desire to learn meteorology and create weather data. I simply want to display existing data in an app. How do I get someone else’s weather data into my app? Well, if someone with weather data like OpenWeatherMap offers an API, which they do, I can make my app ask them to provide it! When we issue a request, it’s called an API Call. When the server issues a response, the meaningful information can be called a Payload. In such a workflow, two things would happen for each call:
- My app issues a call to the OpenWeatherMap API specifying my API key (authentication, more on this later) and that I want the current weather at the latitude and longitude where the app is making the call from.
- OpenWeatherMap sends a response containing a payload of weather conditions at the requested location.
The specific format of the call and what the response will look like can be found in the API documentation. In the example above, I would consult this page of OpenWeatherMap’s documentation.
What does REST mean?
A REST API, also known as a RESTful API is a type of web service API. A web service API mean that URLs are the main way to use the service.
REST itself stands for REpresentational State Transfer. Notably distinct from other types of web service APIs such as SOAP, REST is not a protocol, but instead a set of architectural principles. It’s kind of like how Agile is a set of principles for software development, not methods.
So what are the principles of a REST API?
- Client-server model: RESTful APIs use the client-server model where the client issues requests and the server issues responses.
- Stateless: There is no connection between requests and no client information is stored. Each request must have enough information for the server to process the request.
- Uniform interface: Information must be transferred in a standard form. For our purposes, suffice to say that a REST API will use HTTP, HTTP verbs (GET, PUT, POST, DELETE), resources, and representations of those resources.
- A resource is any “thing” that we can inquire about, such as a slice of pizza or weather data.
- A representation of a resource is what is actually sent in a response. It indicates the state of a resource available to the server. In my pizza analogy, the server did not transfer any slices to us; they only provided information about the state of a slice of cheese pizza. This is the “Representational State” in REST.
- This is not to say that resources cannot be manipulated based on their representation; on the contrary the representation must provide enough information to do so.
- Cacheable: Data must be cacheable to streamline the interaction.
- Layered system: There could be a number of servers between the client issuing the call and the server issuing the response, including those that provide load balancing, security, caching, etc. This needs to be invisible to the client, and cannot affect the request or response.
- Code on demand: Not a requirement for REST, but frequently included in this list. Clients should be able to request code from the server for inclusion in the response, which the client can then execute.
An API must meet each of the criteria above (except code on demand) to be called a REST API. If an API meets the requirements, it can be called “RESTful”.
HTTP Methods
Methods are the verbs you use in an API call to describe what action you want the API to take. HTTP has several commonly used methods: GET, PUT, POST, and DELETE. Others exist, but these are the most common.
- GET: Used to request a representation of a resource. The payload contains the information you request. You don’t use GET to change any information, only to receive it. For example, getting the price of a slice of pizza.
- PUT: Used to update data. For example, giving the pizzeria your new email address to replace your old one on their email list.
- POST: Used to create a brand new resource. For example, sending a brand new order to the pizzeria.
- DELETE: Used to delete information. This one probably doesn’t need an example, does it?
HTTP Response Codes
Each time an HTTP request is made, you are provided with an HTTP status code to indicate whether your request was successful, unsuccessful, or something else happened. There are a lot of status codes, but only a few you need to know without getting into the weeds.
- 200: No problem! Your request was handled without incident.
- 301: The URL of the resource you requested has been moved permanently.
- 404: The server received the response, but no resource exists at the URL requested.
- 500: The server doesn’t know how to handle the request it was given.
Endpoints
An endpoint in terms of a REST API is an exposed URL on the server side where API calls can be sent. It’s where the rubber meets the road; the meeting point between the application issuing calls and the application issuing responses. Perhaps the most helpful way to illustrate this is to refer back to the OpenWeatherMap API documentation.
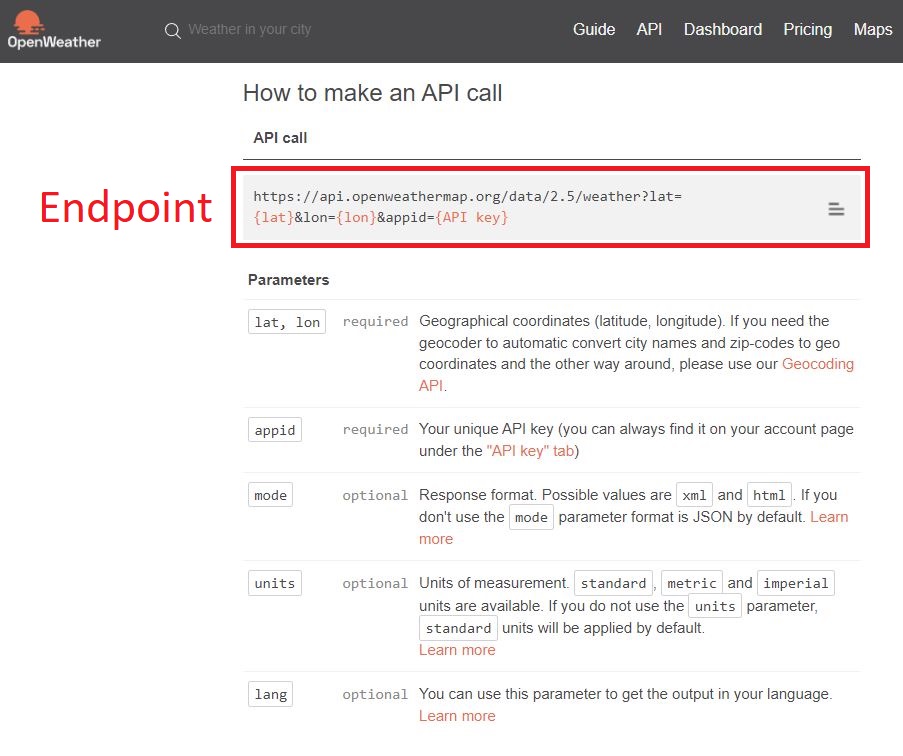
You can see in the image above that the endpoint is comprised of a URL with a variety of parameters that can be altered depending on what you, the requester, need. The server handling API requests knows to expect requests at this endpoint and how to handle the parameters and values provided. In other words, this endpoint has been “exposed” – resources can be retrieved from it.
Note that one of the URL parameters in the screenshot above allows for an API key. If you’re designing an API, don’t do this. I’ll talk more about why when we get to security.
Combine an HTTP method with an endpoint and you almost have a full request. There’s one more component you need: headers.
HTTP Headers
Messages transmitted via HTTP have headers. Think of headers as a way to send additional information alongside the request or response. Each API may use different headers, but there are some common ones to know:
- Content-type: The format in which the response is issued. Most of the time in a REST API, this value will be “application/json”, meaning the response comes in the JSON language.
- Date: The time the message was sent.
- Authentication: Authentication can be handled as a URL parameter passed to the endpoint, but it’s far more secure to handle via HTTP header. It can come in the form of an API access token or a username and password. Documentation should specify which form to use.
Authentication
Since endpoints are accessible to the internet and all of the API specifications are (hopefully) documented, there needs to be some way for a user to be authenticated. Most apps issue access tokens, others issue username and password credentials. If you’re developing an API integration, it’s often advantageous to have test credentials/tokens for developers to use.
Remember, since RESTful APIs are stateless, every request needs to have authentication credentials included.
Securing REST APIs
APIs often have more lax security than, say, the front-end of a web app. It’s important that securing your APIs is not an afterthought. Here are a few practices to keep in mind when auditing your APIs:
- Use HTTPS: With HTTPS, your credentials can be as simply as a randomly generated access token.
- Hash passwords: Where passwords must be used, ensure they are hashed.
- Don’t include sensitive information in the URL parameters: The full URL including parameters is often logged on a web server. The URL itself isn’t hashed, so anyone with access to those logs can see the credentials in plaintext.
- Include time and date in headers: The server handling API calls should time out any requests where the time and date are more than a few seconds out of sync. This prevents simple replay attacks that don’t alter HTTP headers.
- Validate input: Just like any other user input, URL parameters need to be sanitized and validated as soon as possible, before they are processed through the layered system. It may be worth considering an allow list. There are many third-party libraries that can do this, but remember defense-in-depth. Don’t rely on one security feature to keep your API secure.
Conclusion
REST APIs are an excellent, light-weight tool that can greatly enhance your product. You don’t have to be an expert in API development or implementation to understand API documentation. With that knowledge, you can quickly start to see the opportunities applying them to your product.